Tools And Frameworks
Github-best-practices
GitHub - Best Practices for Commits and Branches
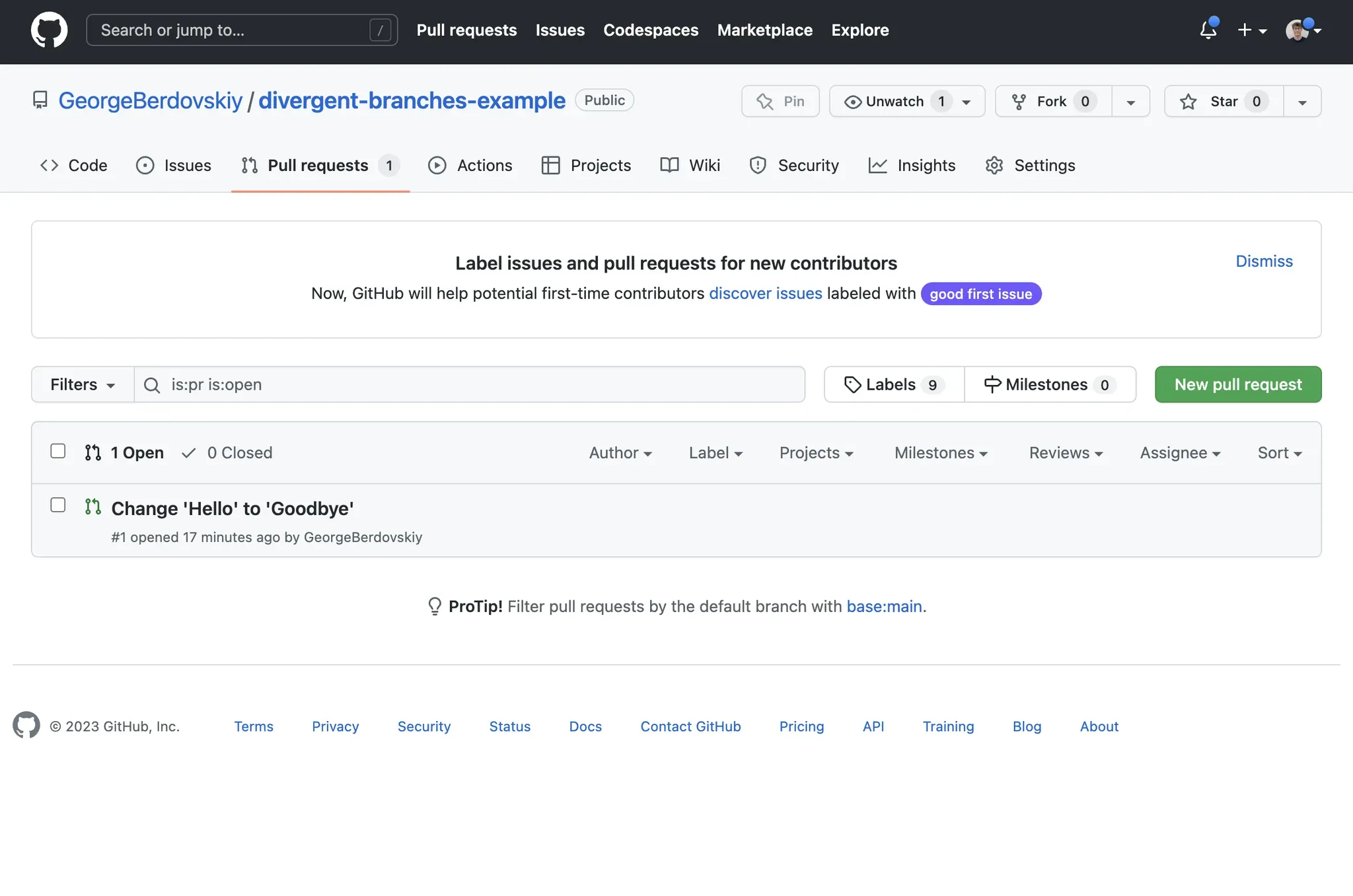
Commits
Commit frequently! But only when nothing is seriously broken. One of the major benefits of Git is the ability to revert your changes if you ever make a mistake or something stops working. If you work for a very long time wihtout committing and need to revert, you’ll lose A LOT of changes. If you commit frequently and need to revert, you won’t lose a lot of changes!
Do not include any files that are unnecessary for the repository or differ between systems. This depends on your project and technology, but this will often include the node modules folder, the build folder, system specific files (such as .DS_Store
files on MacOS), and very often .env files.
The best way to do this is with a .gitignore
file. Before committing, make sure any unwanted files are no longer listed in git status by adding them to your existing .gitignore
file (or create a new one if you don’t already have one).
Include a helpful message that clarifies what was changed in the commit. Try to make sure everything is spelled correctly! It would also be preferable if you followed a consistent capitalization style but that isn’t totally necessary.
Branches
When a feature is ready, you’ll need to merge its branch with your primary branch (development, staged, main, master, or something else). The best way to do this is with a pull request.
On GitHub, press the Pull Requests tab and press New Pull Request. Then, choose which branch you’re merging into which branch, add an informative description of your changes, and press Create Pull Request.
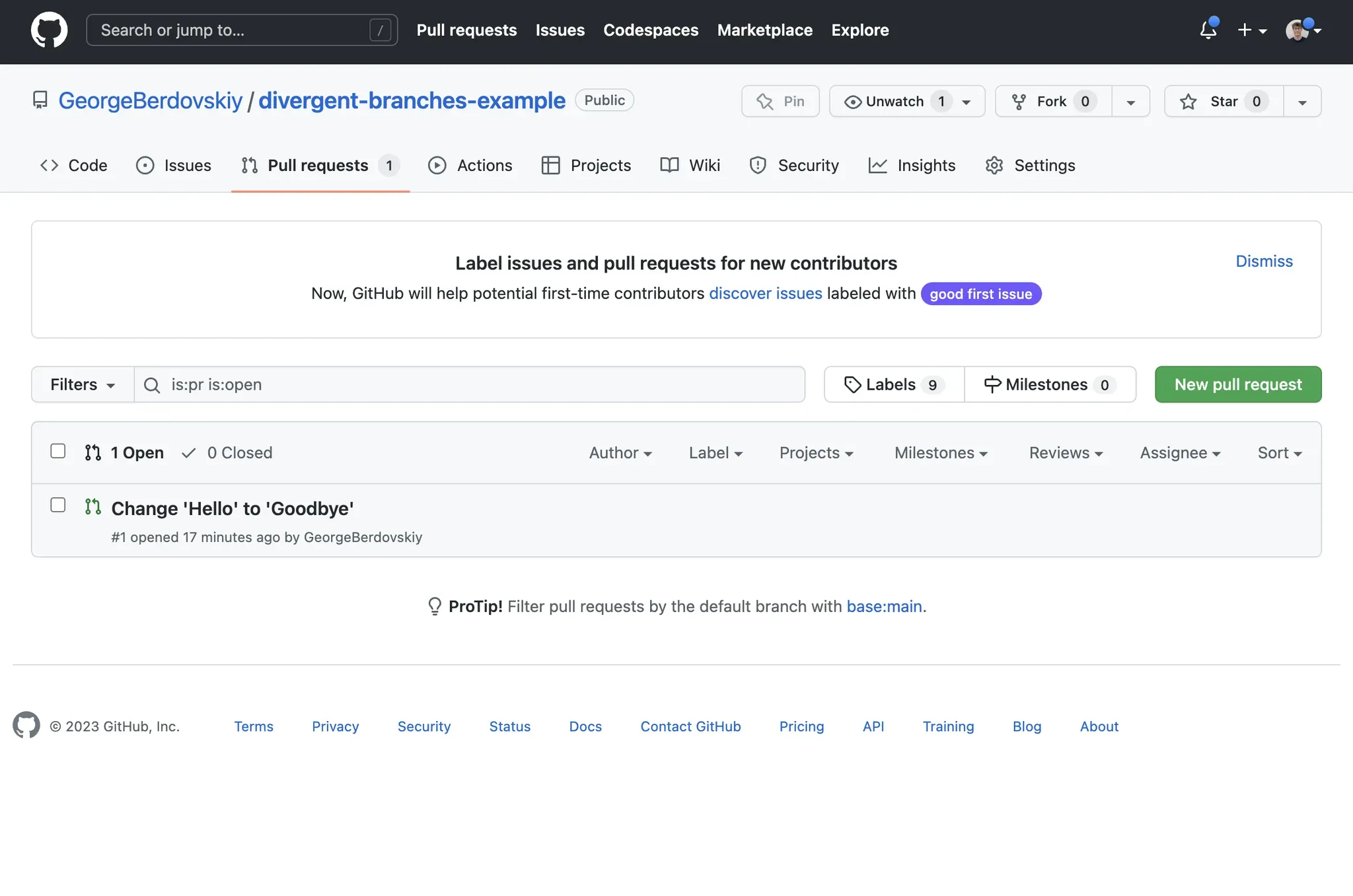
Your resulting pull request will look something like the image below. Notice that you have the ability to view commits and changed files, as well as assign reviewers, contributors, labels, projects, and milestones.
Typically, you’d see a green Merge Pull Request button at the bottom. In this case, it’s greyed out and GitHub tells us that there are conflicts in your branch. Read the next section to see what should be done! It’s easier than you may think.
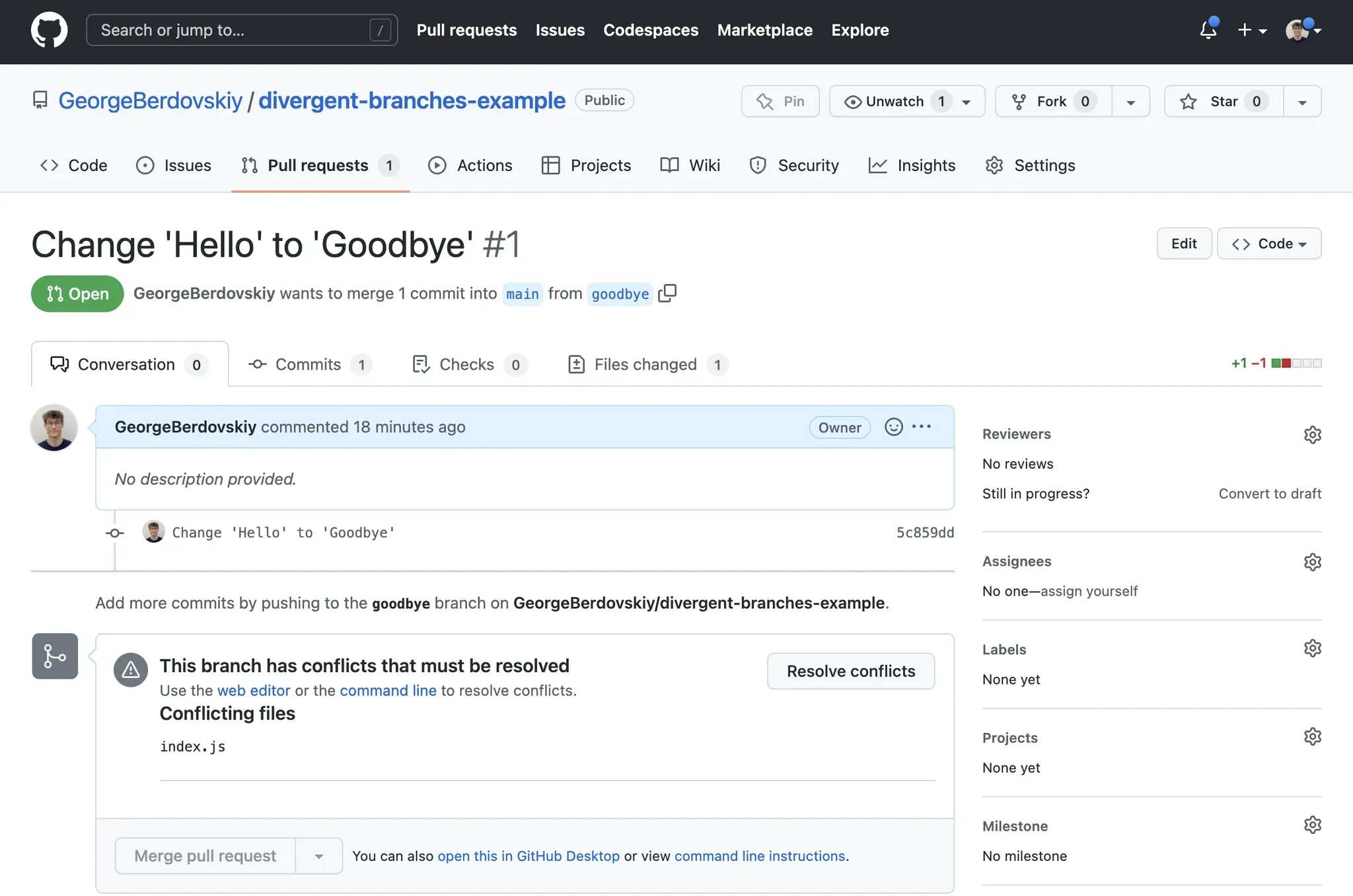
Divergent Branches
OH NO! You tried to pull or merge a pull request and there were some merge conflicts.
Merge conflicts typically occur when you have divergent branches. In other words, after a certain commit, one branch “went” in one direction and another “went” in a different direction. Consider this situation -
- You created branch
main
and commitedindex.js
with the lineconsole.log("Hello!")
- You pushed this change to your remote repository (which could be hosted on GitHub)
- Then you switched to new branch
goodbye
and replaced that line withconsole.log("Goodbye!"
- You pushed this change to a new
goodbye
branch in the same remote repository - You merge branch
goodbye
with branchmain
in your remote repository - Then, you pull from the remote
main
branch while still in your localgoodbye
branch
This will result in a merge conflict, because Git is unsure which line you really want anymore. You’ll typically be given a chance to resolve these conflicts by specifying which changes you want to keep and which ones you want to discard. That will look something like this -
<<<<<<< HEAD
console.log("Goodbye world!")
=======
console.log("What a wonderful world!") // Who wrote this song lyric?
>>>>>>> 7a98254ae6a7a5b55176ff92662847d3a23edefb
In this case, HEAD
is my local change and the other one is the remote. That very long line of characters is the SHA hash of the remote commit I’m trying to merge into my local codebase.
Simply remove all the code and content you don’t want and you’re all set. Here’s what that might look like -
console.log("Goodbye world!")
Finally, you’ll need to commit your changes. Add all changed files, commit, and push as you would normally.
Note that as long as you code carefully and communicate well, you can almost completely avoid merge conflicts! But when working in a large team, it’s not possible to avoid them in all cases. Sometimes programmers will need to change the same code in their own isolated feature branches and that’s OK.
You can do the exact same thing in GitHub when merging branches - you’ll be provided with an editor that has all the files you need to fix.